khan Waseem
Thu Jan 26 2023
-2 min read
What is C?
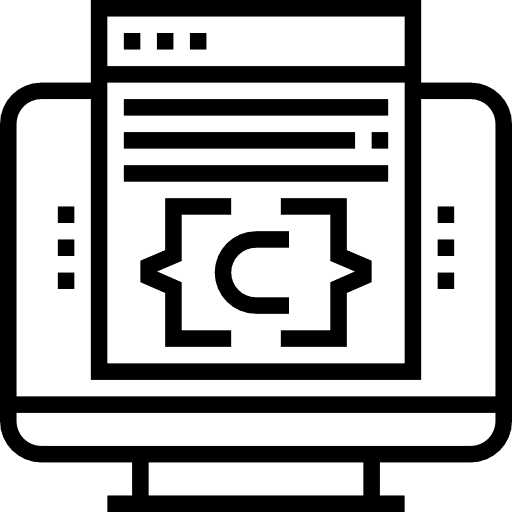
C is a general-purpose, procedural, imperative computer programming language that was first developed in 1972 by Dennis Ritchie at Bell Labs. It is considered a low-level language, as it provides the programmer with direct control over memory and system resources. It is also considered a high-performance language, as it is well-suited for system programming and embedded systems, and it is the basis for many other programming languages, including C++, C#, and Java.
Here is a simple example of a C program that will print “Hello, World!” to the console:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Code language: C/AL (cal)
One of the key features of C is its use of pointers, which allow the programmer to directly manipulate memory addresses. This allows for fine-grained control over the system and can make C programs run faster than programs written in higher-level languages, but also makes it harder to write secure and maintainable code.
Here is an example of using pointers in C to swap the values of two variables:
#include <stdio.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
printf("Before: x = %d, y = %d\n", x, y);
swap(&x, &y);
printf("After: x = %d, y = %d\n", x, y);
return 0;
}
Code language: C/AL (cal)
C is also known for its use of the “top-down” programming model, which begins with a general overview of the problem and then breaks it down into smaller, more manageable pieces. This allows for efficient and structured code organization, but can make it harder to understand the entire program at a glance.
C is often used for system programming tasks such as operating systems and device drivers, as well as embedded systems, such as those found in cars and other devices. It is also commonly used in the development of compilers, interpreters, and other low-level tools.
C is also widely used in the development of open-source software, as it is easy to port to different platforms and has a large and active user community. Some of the most popular open-source software projects, such as the Linux operating system and the Apache web server, are written in C.
Despite its age, C remains a popular programming language today and is still in active use and development. Many modern operating systems, programming languages, and libraries have been written in C, and it continues to be a popular choice for system programming and embedded systems.
C is also considered a low-level language because it provides the programmer with direct control over memory and system resources.