khan Waseem
Fri Jan 27 2023
-4 min read
What is Object Oriented principle?
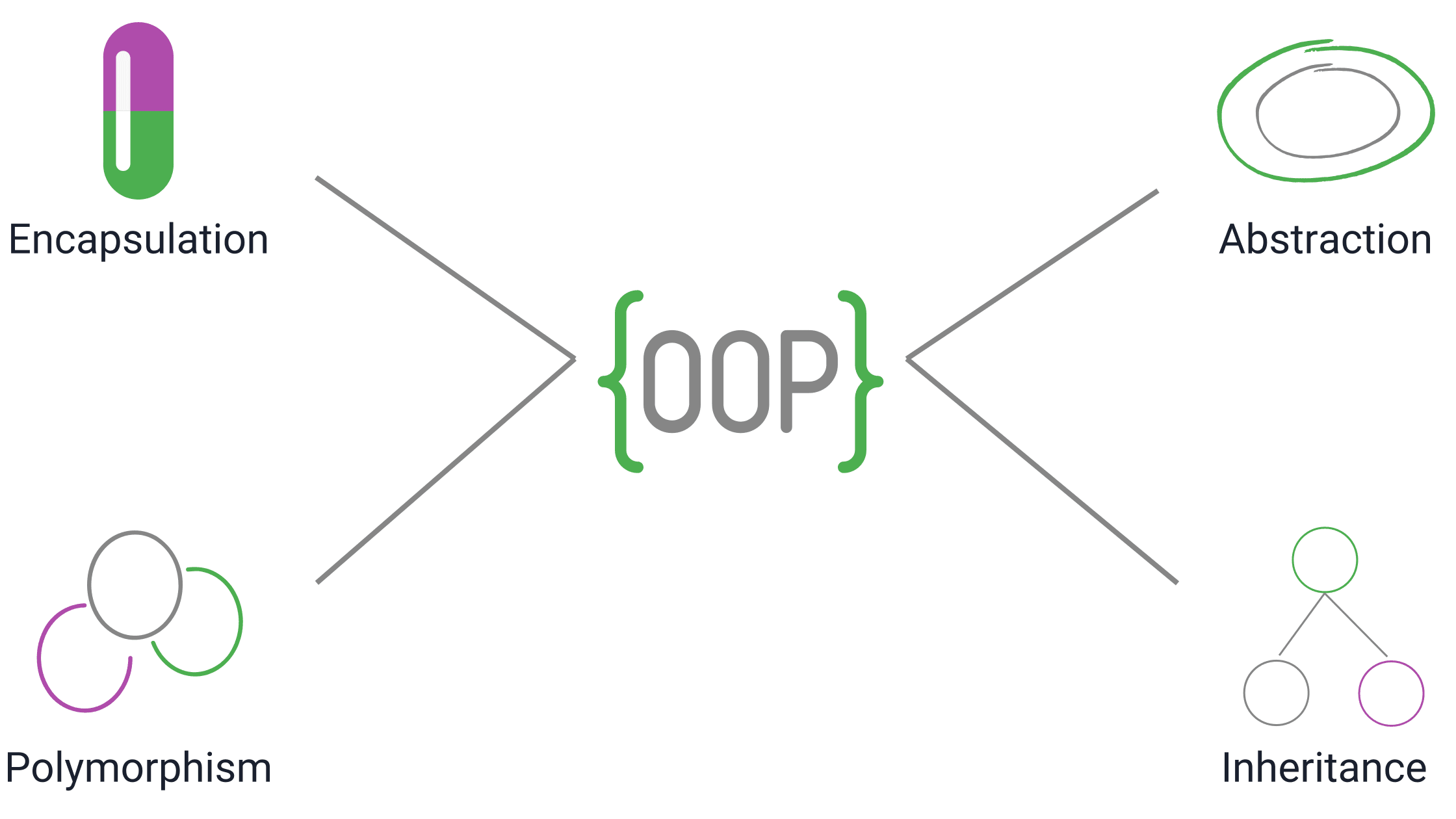
Object-Oriented Programming (OOP) is a programming paradigm that is centered around the concept of “objects.” It provides a way to structure and design software by organizing code into reusable, self-contained units known as objects. These objects represent real-world entities, concepts, or data and encapsulate both data (attributes) and behavior (methods) related to those entities. OOP is based on several key principles and concepts that guide the design and implementation of software systems. In this article, we’ll explore these Object-Oriented principles in detail, covering the essential concepts that define this programming paradigm.
1. Classes and Objects:
At the heart of OOP are classes and objects. A class is a blueprint or template that defines the structure and behavior of objects. It encapsulates the properties (attributes) and methods (functions) that objects of that class will have. Objects, on the other hand, are instances of classes. They represent specificinstances of the real-world entities or concepts that the class describes. For example, a “Car” class can define attributes like “color,” “make,” and “model,” as well as methods like “startEngine” and “drive.”
2. Encapsulation:
Encapsulation is the principle of bundling data (attributes) and the methods (functions) that operate on that data into a single unit, known as an object. This unit hides the internal details of an object’s implementation, exposing only what is necessary for external interaction. Encapsulation helps achieve data hiding, where the internal state of an object is protected from direct external manipulation. This enhances security, as well as the maintainability and reusability of code.
3. Abstraction:
Abstraction is the process of simplifying complex reality by modeling classes based on relevant attributes and behaviors. It allows you to focus on the essential features of an object while ignoring the non-essential details. Abstraction is achieved through classes and interfaces, which provide a high-level view of an object’s capabilities without exposing the intricate implementation details. It helps in managing complexity, making code easier to understand and maintain.
4. Inheritance:
Inheritance is a mechanism that allows a class (called a subclass or derived class) to inherit properties and methods from another class (called a superclass or base class). Inheritance promotes code reuse by allowing you to create a new class that is based on an existing class, inheriting its attributes and behaviors. This enables you to extend or modify the functionality of existing classes without altering their original code. Inheritance also supports the concept of the “is-a” relationship, where a subclass is a specialized version of a superclass.
5. Polymorphism:
Polymorphism refers to the ability of objects of different classes to be treated as objects of a common superclass. It allows you to define methods in the superclass that can be overridden by its subclasses. This way, different subclasses can provide their own implementations for the same method name. Polymorphism allows for more flexible and extensible code, as it enables you to write code that works with objects of various types without knowing their specific implementations.
6. Message Passing:
In OOP, communication between objects is achieved through message passing. When one object wants to interact with another object, it sends a message requesting a particular action. This action can involve method invocation or data retrieval. The receiving object responds by executing the requested action and potentially returning a result. Message passing is a fundamental concept in OOP and supports the idea of collaboration between objects.
7. Cohesion and Coupling:
Cohesion refers to the measure of how closely the responsibilities and functionality within a class are related. High cohesion implies that a class has a well-defined and focused purpose, with its attributes and methods closely aligned to that purpose. Coupling, on the other hand, refers to the degree of interdependence between classes. Low coupling indicates that classes are loosely connected, reducing the impact of changes in one class on other classes. Achieving high cohesion and low coupling enhances code quality and maintainability.
8. Association, Aggregation, and Composition:
Association, aggregation, and composition are relationships between classes that define how objects interact and collaborate. Association represents a generic link between classes, indicating that objects of one class are related to objects of another class. Aggregation represents a “whole-part” relationship, where one class (the whole) is composed of or contains instances of another class (the parts). Composition is a stronger form of aggregation, implying a close relationship where the parts cannot exist without the whole.
9. SOLID Principles:
The SOLID principles, introduced earlier, are a set of five guidelines that promote good software design in object-oriented programming. They include the Single Responsibility Principle (SRP), Open/Closed Principle (OCP), Liskov Substitution Principle (LSP), Interface Segregation Principle (ISP), and Dependency Inversion Principle (DIP). These principles provide best practices for creating maintainable, flexible, and robust software systems. Read More
In conclusion, Object-Oriented Programming (OOP) is a powerful programming paradigm that emphasizes the organization of code into reusable and self-contained objects. These objects encapsulate data and behavior, allowing for a more intuitive representation of real-world entities and concepts in software systems. Key OOP principles include classes and objects, encapsulation, abstraction, inheritance, polymorphism, message passing, cohesion, and coupling. By applying these principles, developers can create software that is modular, maintainable, and adaptable to changing requirements, making OOP a fundamental approach in modern software development.