khan Waseem
Fri Jan 27 2023
-3 min read
Sorting Algorithms
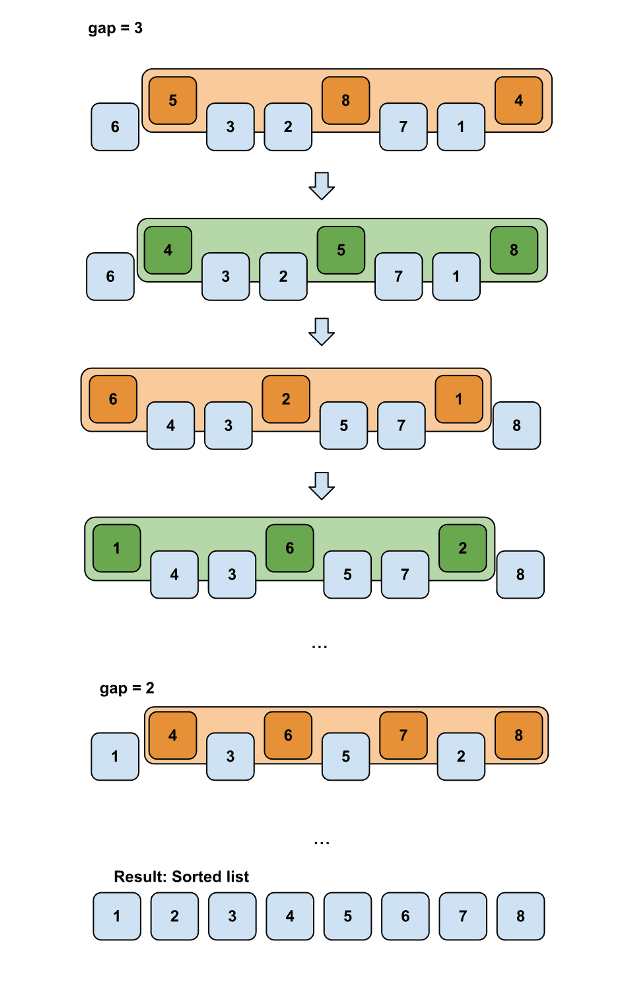
Sorting Algorithms: Organizing Chaos into Order
In the realm of computer science, sorting algorithms stand as fundamental tools for arranging elements in a specific order. Sorting is a foundational operation that finds applications in various domains, from database management to data analysis and beyond. A well-designed sorting algorithm can significantly impact the efficiency and responsiveness of software systems. This comprehensive exploration delves into the intricacies of sorting algorithms, their types, working principles, efficiency considerations, and real-world applications.
The Significance of Sorting Algorithms
Sorting is the process of arranging elements in a specific order, such as ascending or descending. This operation is essential for tasks that require data to be organized for efficient retrieval, comparison, and analysis. Sorting algorithms provide the structure and predictability needed to make data manipulation tasks feasible and reliable.
Types of Sorting Algorithms
Sorting algorithms can be categorized into various types based on their approach to sorting:
Comparison-Based Sorting Algorithms: These algorithms compare elements using comparison operations and then reorder them accordingly. Examples include Bubble Sort, Insertion Sort, Selection Sort, Merge Sort, Quicksort, and Heap Sort.
Non-Comparison Sorting Algorithms: These algorithms do not rely on direct element comparisons. Instead, they utilize unique properties of the elements to determine their order. Examples include Counting Sort, Radix Sort, and Bucket Sort.
Working Principles of Sorting Algorithms
Comparison-Based Sorting Algorithms:
Bubble Sort: This simple algorithm repeatedly compares adjacent elements and swaps them if they are in the wrong order. The process continues until the entire array is sorted.
Insertion Sort: Elements are iteratively picked and inserted into their correct positions in the already sorted part of the array.
Selection Sort: The smallest (or largest) element is found and swapped with the first (or last) element. This process is repeated for the remaining unsorted part of the array.
Merge Sort: This divide-and-conquer algorithm divides the array into smaller subarrays, sorts them, and then merges them to achieve the final sorted array.
Quicksort: Also a divide-and-conquer algorithm, Quicksort chooses a pivot element and partitions the array into elements smaller and larger than the pivot. These subarrays are then recursively sorted.
Heap Sort: Elements are treated as a binary heap and repeatedly extracted and inserted into their correct positions in a separate sorted array.
Non-Comparison Sorting Algorithms:
Counting Sort: This algorithm works for datasets with a limited range of values. It counts the occurrences of each value and constructs a sorted output array.
Radix Sort: Radix Sort processes elements digit by digit, distributing elements into buckets based on their digits.
Bucket Sort: Elements are divided into buckets and each bucket is sorted individually, then combined to obtain the final sorted array.
Efficiency Considerations
The efficiency of sorting algorithms is often measured in terms of time complexity, which indicates how the algorithm’s performance scales with the size of the dataset.
Comparison-Based Sorting Algorithms:
Bubble Sort: Worst-case and average-case time complexity is O(n^2).
Insertion Sort: Worst-case and average-case time complexity is O(n^2).
Selection Sort: Worst-case and average-case time complexity is O(n^2).
Merge Sort: Best, worst, and average-case time complexity is O(n log n).
Quicksort: Best-case time complexity is O(n log n), while worst and average-case time complexity is O(n^2).
Heap Sort: Worst-case and average-case time complexity is O(n log n).
Non-Comparison Sorting Algorithms:
Counting Sort: Time complexity is O(n + k), where k is the range of values.
Radix Sort: Time complexity is O(d * (n + k)), where d is the number of digits and k is the range of values.
Bucket Sort: Time complexity varies based on the sorting algorithm used within each bucket.
Real-World Applications
Sorting algorithms find applications in numerous domains:
Database Management: Sorting is essential for maintaining efficient data retrieval and manipulation in databases.
Search Engines: Search engines use sorting to rank search results based on relevance.
E-commerce: Online stores use sorting to arrange products based on various criteria like price or popularity.
Data Analysis: Sorting facilitates the analysis of data by presenting it in an organized and easily interpretable format.
Graphics: Rendering objects in graphical applications requires sorting to determine the order in which they are displayed.
Music and Video Players: Sorting algorithms help organize playlists and media libraries.
Conclusion
Sorting algorithms are the backbone of data organization and manipulation. They are fundamental tools in computer science, enabling efficient retrieval, comparison, and analysis of data. By understanding the different types of sorting algorithms, their working principles, efficiency considerations, and real-world applications, developers can make informed decisions about which algorithm to use based on the characteristics of the dataset and the desired performance outcomes. Sorting algorithms empower software systems to process and present data in a structured and meaningful way, contributing to the efficiency and functionality of various applications.